Introduction to React Hooks
Hooks are a new addition in React 16.8. With Hooks, you can utilize state and other React features without writing a class. React Hooks were introduced at React Conf in October 2018 as a means to use state and side-effects (see lifecycle methods in class components) in React function components. Earlier, function components have been called functional stateless components (FSC), but now it’s possible to use state with React Hooks and hence many people mention them as function components today.
BLOGS
- Posted 2 years ago

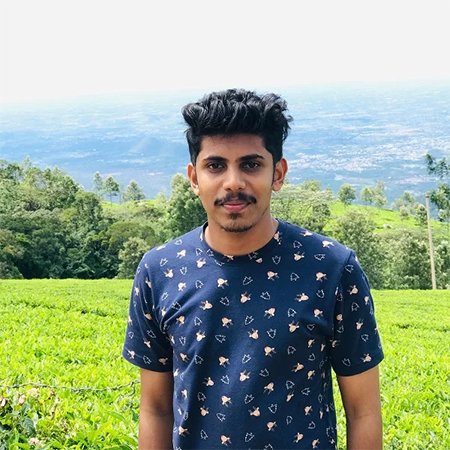
By Rafi CH (Senior Lead Developer)
- 2 years ago
Introduction to React Hooks
Hooks are a new addition in React 16.8. With Hooks, you can utilize state and other React features without writing a class. React Hooks were introduced at React Conf in October 2018 as a means to use state and side-effects (see lifecycle methods in class components) in React function components. Earlier, function components have been called functional stateless components (FSC), but now it’s possible to use state with React Hooks and hence many people mention them as function components today.
WHY REACT HOOKS?
React Hooks allow us to compose React applications with only function components. If you write a function component, and later you want to add some state to it, the only way you can handle this was by converting it to a class. But, the same is feasible now by using a Hook inside the existing function component.
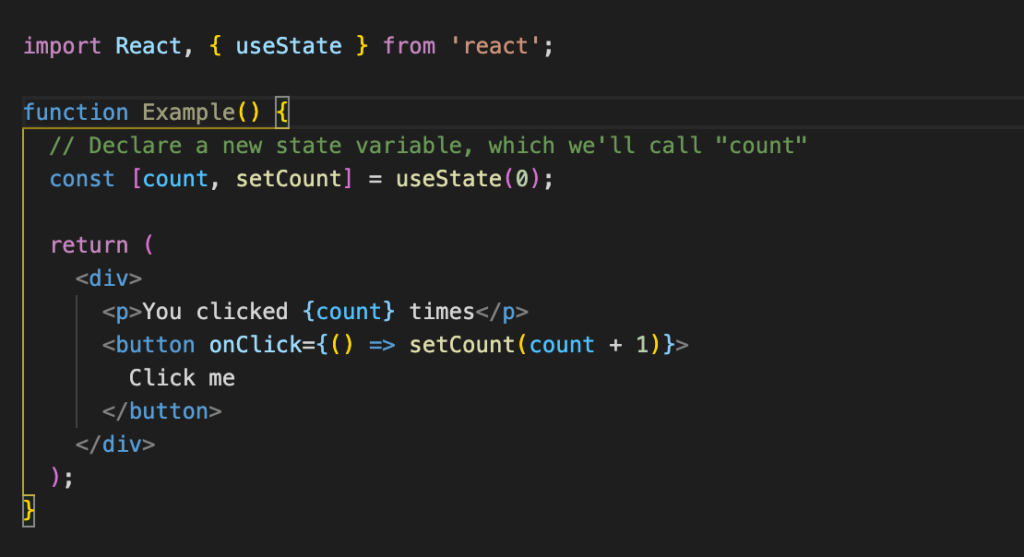
RULES OF HOOKS
Hooks are comparable to JavaScript functions. Hooks rule assures that all the stateful logic in a component is visible in its source code. These rules are:
Only call Hooks at the top level
Hooks must be used at the top level of the React functions. Avoid the practice of calling Hooks inside loops, conditions, or nested functions. This rule guarantees that Hooks are called in the same order each time a component renders.
Only call Hooks from React functions
It’s not possible to call Hooks from regular JavaScript functions. Rather, you can call Hooks from React function components. Also, Hooks can be called from custom Hooks.
REACT USESTATE HOOK
Hook state is the new method of declaring a state in React app. Hook utilizes useState() functional component for setting and retrieving state.
//Declare a new state variable, which we’ll call”count”
const [count, setCount] = useState(0);
const [count, setCount] = useState(0);
The useState is the Hook that needs to call inside a function component to add some local state to it. The useState returns a pair where the first element is the current state value/initial value, and the second one is a function that enables us to update it. After that, we will call this function from an event handler or someplace else. The useState is similar to this.setState in class.
REACT USEEFFECT HOOK
The Effect Hook useEffect() allows you to perform side effects in function components. It does not use components lifecycle methods that are present in class components. In other words, Effects Hooks are similar to componentDidMount(), componentDidUpdate(), and componentWillUnmount() lifecycle methods.
Side effects have common features in which most web applications need to operate, such as:
- Updating the DOM
- Fetching and consuming data from a server API
- Setting up a subscription, etc.
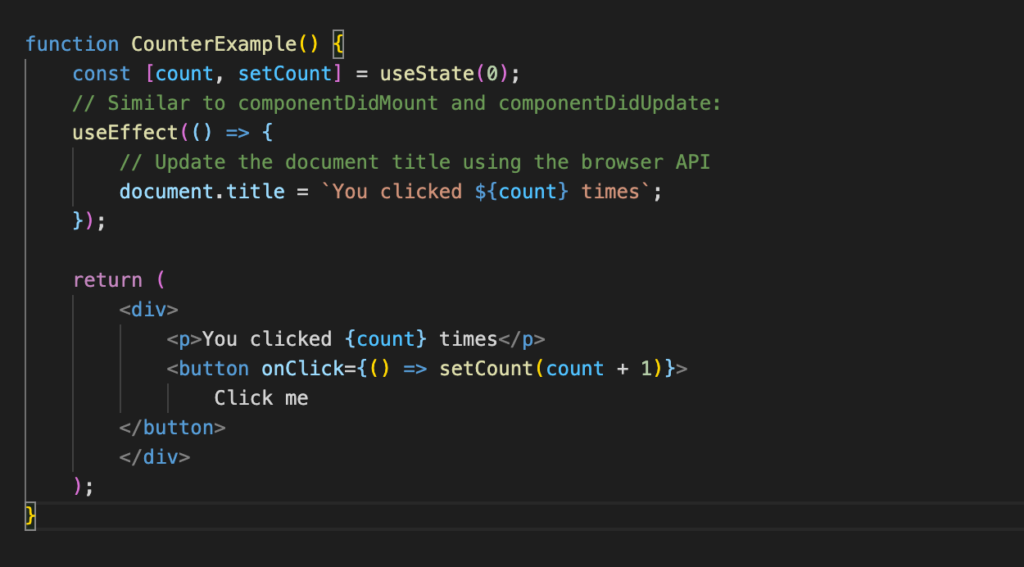
The above code is an illustration in which we set the document title to a custom message, including the number of clicks.
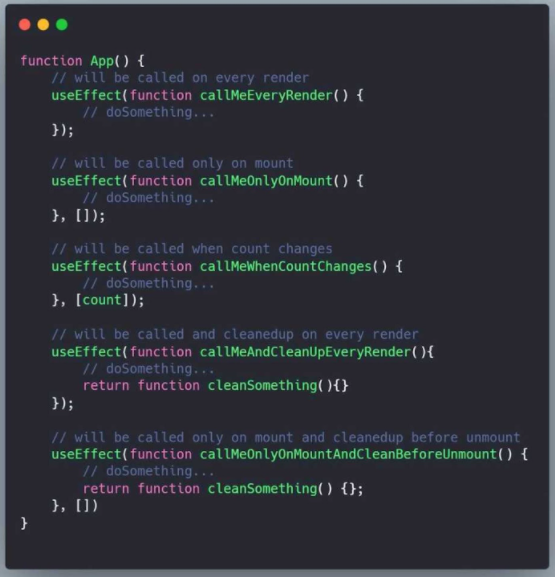
REACT BUILT-IN HOOKS
Only call Hooks at the top level
- useState
- useEffect
- useContext
Additional Hooks
- useReducer
- useCallback
- useMemo
- useRef
- useImperativeHandle
- useLayoutEffect
- useDebugValue
CONCLUSION
Hooks improve the design pattern of our code and the performance of our app, and it is recommendable to use the same in your web app development projects. It simplifies the code by forcing us to avoid the usage of some of the ceremonies while defining components. And thereby making the code cleaner and readable. Class Components will not go outdated and hence one doesn’t need to edit Class Based Components using Hooks.